LLM Proxies: Friend or Foe?
Dive into the trade-offs of using LLM proxies in your LLM Ops workflow. Explore the benefits, downsides, and why a proxyless approach might be better.
LLM proxies have emerged as a seemingly convenient tool in the LLM Ops workflow, offering features like caching, rate limiting, and a unified API for multiple LLM providers. While they initially appear beneficial, a closer look reveals significant drawbacks that can hinder your application's performance, scalability, and reliability. This post delves into the technical nuances of LLM proxies, exploring their advantages and disadvantages, and ultimately arguing for a proxyless approach augmented by robust observability tools.
typescript
What Are LLM Proxies?
An LLM proxy sits between your application and the LLM provider (e.g., OpenAI, Hugging Face). It intercepts requests and responses, acting as a middleware layer:
User -> Your Application -> LLM Proxy -> LLM Provider -> LLM Proxy -> Your Application -> User
Generally, by inserting themselves as an intermediary, LLM proxies offer additional functionality and control over the LLM provider's API. This includes features like caching, rate limiting, request routing, and key management, which can help optimize your LLM workflows. They also allow you to interact with multiple LLM models through a single interface, simplifying integration and management.
However, LLM proxies come with trade-offs that can impact your application's performance, reliability, and security by introducing synchronous latency for features that would better be handled asynchronously, as well as creating a single point of failure, and limiting visibility into LLM operations. Most, if not all, proxies are also prone to lag behind the rapid evolution of LLM provider APIs, potentially delaying access to new features and models.
Is the convenience of LLM proxies worth the risks? Let's explore the pros and cons in more detail.
Benefits of Using LLM Proxies
- Caching: Store and reuse LLM responses, reducing API calls and latency for repeated queries. This can significantly impact cost, especially for frequently used prompts.
- Rate Limiting: Control the rate of requests to LLM providers, preventing you from exceeding quotas and incurring unexpected costs. This can also smooth out bursts of traffic and improve the overall stability of your application.
- Unified API: Abstract away the complexities of different LLM provider APIs, providing a single, consistent interface for interacting with multiple models. This simplifies integration and allows for easier switching between providers.
- Request Routing: Dynamically route requests to different models based on criteria like cost, performance, or specific capabilities. This enables optimization for various use cases within a single application.
- Key Management: Securely manage and rotate API keys for different LLM providers without exposing them directly in your application code.
Downsides of Using LLM Proxies
- Latency: Introducing an extra hop in the request path inevitably adds latency, impacting the responsiveness of your application, especially noticeable in real-time or interactive applications.
- Single Point of Failure: The proxy becomes a critical component in your infrastructure. If it fails, your entire application's LLM functionality goes down. This risk outweighs many of the perceived benefits.
- Security & Privacy Concerns: All your LLM traffic flows through the proxy, potentially exposing sensitive data if the proxy is not adequately secured. Choosing a trusted provider and implementing robust security measures becomes paramount.
- Limited Visibility & Debugging: Proxies often obscure the true source of errors and performance bottlenecks. Debugging can become more complex as you need to consider both the application, the proxy itself, and the LLM provider.
- Vendor Lock-in: Adopting a specific proxy can tie you to its features and limitations. Switching to a different proxy or a proxyless architecture later can require significant code changes.
- API Lag: Proxies often lag behind the rapid evolution of LLM provider APIs. New features and models might not be immediately available through the proxy, slowing down your development cycle.
Why Proxyless LLM is Better
A proxyless architecture, combined with a dedicated LLM observability platform (like Modelmetry or Langsmith), offers a more robust and flexible solution.
By directly integrating with the LLM provider and instrumenting your code with tracing and logging, you gain:
- Latest Features: Access new models and features from LLM providers as soon as they are released, without waiting for proxy updates.
- Reduced Latency: Eliminate the extra hop introduced by the proxy, improving application responsiveness.
- Improved Reliability: Avoid creating a single point of failure. Your application interacts directly with the LLM provider, minimizing dependencies.
- Enhanced Observability: Gain deep insights into LLM performance, including detailed traces, latency breakdowns, and error analysis. This helps identify and address bottlenecks quickly.
- Flexibility and Control: Maintain full control over your LLM integration and leverage the latest features and models from providers without waiting for proxy updates.
- Simplified Debugging: Directly interact with the LLM provider APIs, making it easier to pinpoint the source of issues and debug effectively.
typescript
As you can see, it's a bit more verbose but you can then use a dedicated observability platform to monitor and trace your LLM workflows effectively. With Modelmetry, you can enjoy a no-code setup, detailed insights, and real-time monitoring of your LLM applications.
Alternatives to Proxies: Frameworks & Direct Integrations
Frameworks and platforms like LangChain and LlamaIndex offer a structured approach to LLM application development, providing abstractions and utilities for common tasks without introducing a proxy layer.
Directly integrating with the provider's API using their official SDKs gives you maximum control and access to the latest features. Combine this with a dedicated observability platform to gain comprehensive monitoring and tracing without the drawbacks of a proxy.
Observability & Guardrails Without An LLM Proxy
Implementing robust observability tools and guardrails in your LLM application can help mitigate many of the risks associated with a proxyless approach. By instrumenting your code with detailed logging, tracing, and monitoring, you can ensure reliable performance, identify issues quickly, and maintain a high level of visibility into your LLM workflows.
Whilst instrumenting your code may seem daunting, tools like Modelmetry and Langsmith provide easy-to-use SDKs and integrations that streamline the process. By investing in observability early, you can build a more resilient and scalable LLM application that meets your performance and reliability requirements.
You can check out Modelmetry's SDK documentation and Langsmith's integration guide for more information on how to get started with observability in your LLM applications.
Conclusion
While LLM proxies offer some initial conveniences, their limitations and potential risks often outweigh the benefits, especially for production-grade applications. A proxyless approach, complemented by robust observability and well-structured frameworks, provides a more scalable, reliable, and performant solution for building and managing LLM-powered applications. Embrace the direct approach for greater control, flexibility, and ultimately, a better user experience.
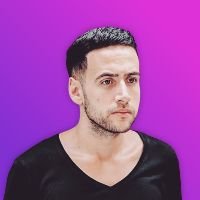
Lazhar Ichir
Lazhar Ichir is the CEO and founder of Modelmetry, an LLM guardrails and observability platform that helps developers build secure and reliable modern LLM-powered applications.